Every Django Application has the magnificent Django Admin built in enabling you to access your database tables in a very nice way.
The url is typically admin/
The problem is that hackers also know the default admin url for a Django install is admin/
So what do you do? Well, you change the admin url in your base urls.py file
# Default admin url
# path('admin/', admin.site.urls),
# New super secret admin url!
path('secret_admin_url/', admin.site.urls),
But sometimes that is not enough.
The above will hide your admin (you are picking a better name than secret_admin_url aren’t you?) but sometimes it’s useful to know who is trying to access your admin.
Enter the plugin django-admin-honeypot
Django Admin Honeypot creates an admin page for you that looks exactly like the real one. Its url is admin/ and it advises you to create a secret admin url that becomes your real admin.
Installation is simple:
pip install django-admin-honeypot
Then add it to installed apps:
INSTALLED_APPS = (
...
'admin_honeypot',
...
)
Then update your base urls.py file:
urlpatterns = patterns(''
...
url(r'^admin/', include('admin_honeypot.urls', namespace='admin_honeypot')),
url(r'^secret_admin_url/', include(admin.site.urls)),
...
)
Then do a migrate:
python manage.py migrate
When hackers try to login to the default admin/ page – they won’t be successful.
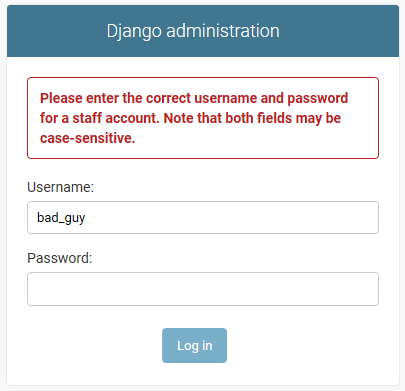
The login attempt will be logged in your real admin.

From there you can attend to the probe as you wish.
Conclusion
So while a honeypot like this is not a perfect solution to securing your Django website – it is an excellent step to ensure your admin is not vulnerable to being probed.